A Beginners Guide to Ruby Code Golf
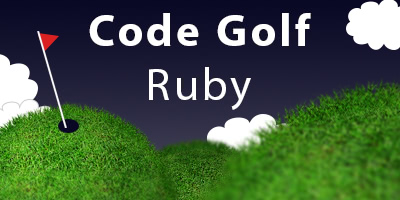
This article, inspired by a similar tutorial about Rust, tries to go over the main features of Ruby code golf.
Code Golf is a novelty to create the same functionality in a few characters as possible. This help learn the ins and outs of a language and forces you to think outside the box. It’s not very practical for production code, but it’s super fun!
Mass Assignment
a,b=5,6
Use Less Than instead of Equals
a%2==0
a%2<1
Use print, puts, or p
print
puts
p
Create Arrays Using the Shortcut Notation %w
You can create arrays of strings by using the following notation:
a = %w(a b c) => ["a","b","c"]
Ternary Operator for Logic
Statement ? True Condition : False Condition
Example:
a>10?"big":"small"
Scientific Notation for Numbers
a=1000000
b=1e6
a==b # true
Tricks with «
a.push x
# becomes
a<<x
Some math tricks
(i - 1) * 2
# can be written as
~-i * 2
Cloning a string
a="string"
b=a.clone
b=a.dup
b=+-a
b=a*1
b=-a
Read from `dd` instead of gets
a,*d=`dd`.split.map &:to_i
p d.min+d.max
Using eval
While array.sum is great and shorter than array.reduce(:+), there are no similar methods for the other operators *, -, / …
Joining arrays and evaling them saves some space:
a = [1,2,3,4]
a.reduce(:*) # => 24
eval(a*?*) # => 24
It also removes the need to map string input to numbers first:
b = "1 2 3 4".split
b.map(&:to_i).reduce(:*)
eval(b*?*)
Predefined Variables
There are a lot of predefined variables we can use. Here’s a few examples.
# While there are lines to get read the lines
while gets
puts $_
x,y,z=gets.split.map &:to_i
# Or written as
$/=' '
x,y,z=$<.map &:to_i
Variable | Description |
---|---|
$! |
The last exception object raised. The exception object can also be accessed using => in rescue clause. |
$@ |
The stack backtrace for the last exception raised. The stack backtrace information can retrieved by Exception#backtrace method of the last exception. |
$/ |
The input record separator (newline by default). gets, readline, etc., take their input record separator as optional argument. |
$\ |
The output record separator (nil by default). |
$, |
The output separator between the arguments to print and Array#join (nil by default). You can specify separator explicitly to Array#join. |
$; |
The default separator for split (nil by default). You can specify separator explicitly for String#split. |
$. |
The number of the last line read from the current input file. Equivalent to ARGF.lineno. |
$< |
Synonym for ARGF. |
$> |
Synonym for $defout. |
$0 |
The name of the current Ruby program being executed. |
$$ |
The process pid of the current Ruby program being executed. |
$? |
The exit status of the last process terminated. |
$defout |
The destination output for print and printf ($stdout by default). |
$F |
Receives the output from split when -a is specified. This variable is set if the -a command-line option is specified along with the -p or -n option. |
$stdin |
Standard input (STDIN by default). |
$stdout |
Standard output (STDOUT by default). |
$stderr |
Standard error (STDERR by default). |
$VERBOSE |
True if the -v, -w, or –verbose command-line option is specified. |
$- x |
The value of interpreter option -x (x=0, a, d, F, i, K, l, p, v). These options are listed below |
$-0 |
The value of interpreter option -x and alias of $/. |
$-a |
The value of interpreter option -x and true if option -a is set. Read-only. |
$-d |
The value of interpreter option -x and alias of $DEBUG |
$-F |
The value of interpreter option -x and alias of $;. |
$-i |
The value of interpreter option -x and in in-place-edit mode, holds the extension, otherwise nil. Can enable or disable in-place-edit mode. |
$-I |
The value of interpreter option -x and alias of $:. |
$-l |
The value of interpreter option -x and true if option -lis set. Read-only. |
$-p |
The value of interpreter option -x and true if option -pis set. Read-only. |
$_ |
The local variable, last string read by gets or readline in the current scope. |
$~ |
The local variable, MatchData relating to the last match. Regex#match method returns the last match information. |
$n |
The string matched in the nth group of the last pattern match. Equivalent to m[n], where m is a MatchData object. |
$& |
The string matched in the last pattern match. Equivalent to m[0], where m is a MatchData object. |
$` |
The string preceding the match in the last pattern match. Equivalent to m.pre_match, where m is a MatchData object. |
$' |
The string following the match in the last pattern match. Equivalent to m.post_match, where m is a MatchData object. |
$+ |
The string corresponding to the last successfully matched group in the last pattern match. |
More links for code golf in ruby Stack Exchange